When I first started working with Scala in VS Code, one of the most frustrating challenges was getting my code to format consistently. Scala’s syntax is expressive and concise, but without a proper formatter, it can easily become messy and difficult to read. After some trial and error, I discovered the right way to set up code formatting for Scala using Scalafmt and Metals. In this post, I’ll walk you through the steps I took to ensure my Scala code stays clean and professional.
Thank me by sharing on Twitter 🙏
Why Formatting Scala Matters
Before diving into the technical setup, I want to touch on why code formatting is so important. Consistent formatting isn’t just about aesthetics—it’s about readability, maintainability, and team collaboration. A well-formatted codebase reduces cognitive load and helps developers focus on solving problems rather than deciphering syntax. With a reliable formatter in place, you can let the tool handle the nitty-gritty details of spacing, indentation, and alignment while you focus on writing meaningful code.
Setting Up Metals in VS Code
The first step to enabling Scala code formatting in VS Code is to install the Metals extension. Metals is the go-to Scala language server for VS Code, providing robust support for features like autocompletion, syntax highlighting, and code navigation.
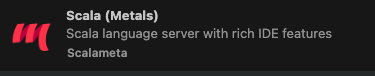
Here’s how I set it up:
- Open VS Code and navigate to the Extensions view by pressing
Ctrl+Shift+X
(Windows/Linux) orCmd+Shift+X
(Mac). - In the search bar, type “Metals” and install the Scala (Metals) extension from Scalameta.
- Once installed, open a Scala project, and Metals will automatically prompt you to import the build. Follow the instructions to complete the setup.
At this point, Metals is ready to provide language server functionality, but we still need to configure Scalafmt for formatting.
The Nvidia Way: Jensen Huang and the Making of a Tech Giant
$26.16 (as of December 21, 2024 19:39 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)HP 67 Black/Tri-color Ink Cartridges (2 Pack) | Works with HP DeskJet 1255, 2700, 4100 Series, HP ENVY 6000, 6400 Series | Eligible for Instant Ink | 3YP29AN
$36.89 (as of December 21, 2024 08:38 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Elon Musk
$22.96 (as of December 21, 2024 19:39 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Installing and Configuring Scalafmt
Scalafmt is a popular tool for formatting Scala code. It ensures that your code adheres to a consistent style, making it easier to read and review.
Adding Scalafmt to Your Project
To use Scalafmt, it needs to be integrated into your Scala project. Here’s what I did:
- Open the
project/plugins.sbt
file and add the following line:addSbtPlugin("org.scalameta" % "sbt-scalafmt" % "2.5.0")
This installs the Scalafmt plugin for SBT, Scala’s build tool. - In your
build.sbt
file, enable Scalafmt formatting on compile:ThisBuild / scalafmtOnCompile := true
- Run the following command in your terminal to apply Scalafmt for the first time:
sbt scalafmt
Creating a Scalafmt Configuration
To customize Scalafmt’s behavior, you’ll need a .scalafmt.conf
file in your project’s root directory. Here’s an example configuration I often start with:
version = "3.7.1"
maxColumn = 100
align = none
style = default
This configuration limits line length to 100 characters, disables alignment, and uses the default formatting style. You can tweak these settings to match your team’s preferences.
Enabling Formatting in VS Code
With Metals and Scalafmt set up, the next step is to configure VS Code to automatically format your Scala code on save. This step ties everything together and ensures that you don’t have to run Scalafmt manually each time.
- Open the VS Code settings by pressing
Ctrl+,
(Windows/Linux) orCmd+,
(Mac). - In the settings search bar, type “default formatter.”
- For the Editor: Default Formatter setting, select
scalameta.metals
from the dropdown. This tells VS Code to use Metals as the default formatter for Scala files. - To enable format-on-save, add the following to your
settings.json
file:{ "editor.formatOnSave": true, "[scala]": { "editor.defaultFormatter": "scalameta.metals" } }
Now, every time you save a Scala file, VS Code will use Scalafmt to reformat it automatically.
Testing Your Setup
At this point, your setup should be ready to go. To confirm that everything works as expected:
- Open a Scala file in your project.
- Write some poorly formatted code, for example:
object Example{ def main(args:Array[String]) {println("Hello, World!")}}
- Save the file (
Ctrl+S
orCmd+S
), and watch as it’s reformatted to:object Example { def main(args: Array[String]): Unit = { println("Hello, World!") } }
This quick test ensures that Scalafmt and Metals are working together correctly in VS Code.
Formatting an Entire Project
Sometimes, you might want to reformat all the Scala files in your project. This is especially useful when integrating Scalafmt into an existing codebase for the first time.
To format all files, run the following command in your terminal:
sbt scalafmtAll
This command applies Scalafmt to every Scala file in your project, ensuring consistent formatting across the board.
Troubleshooting Common Issues
While setting up code formatting, I encountered a few bumps along the way. Here are some common problems and how I solved them:
- Scalafmt not formatting on save: Make sure the
scalameta.metals
formatter is selected in VS Code’s settings and that format-on-save is enabled insettings.json
. - Build import issues with Metals: If Metals fails to import your project, try running
sbt clean
and restarting VS Code. - Invalid Scalafmt configuration: Double-check your
.scalafmt.conf
file for syntax errors. The Metals output window often provides helpful error messages if the configuration is invalid.
By addressing these issues early, I was able to get my formatter working smoothly.
Wrapping Up
Setting up code formatting for Scala in VS Code might seem like a lot of effort initially, but once everything is in place, it becomes a seamless part of your workflow. With Scalafmt handling the formatting and Metals providing robust language support, you can focus on writing quality Scala code without worrying about the small details. A consistent and clean codebase is not just a technical achievement—it’s a step toward better collaboration and more maintainable software.